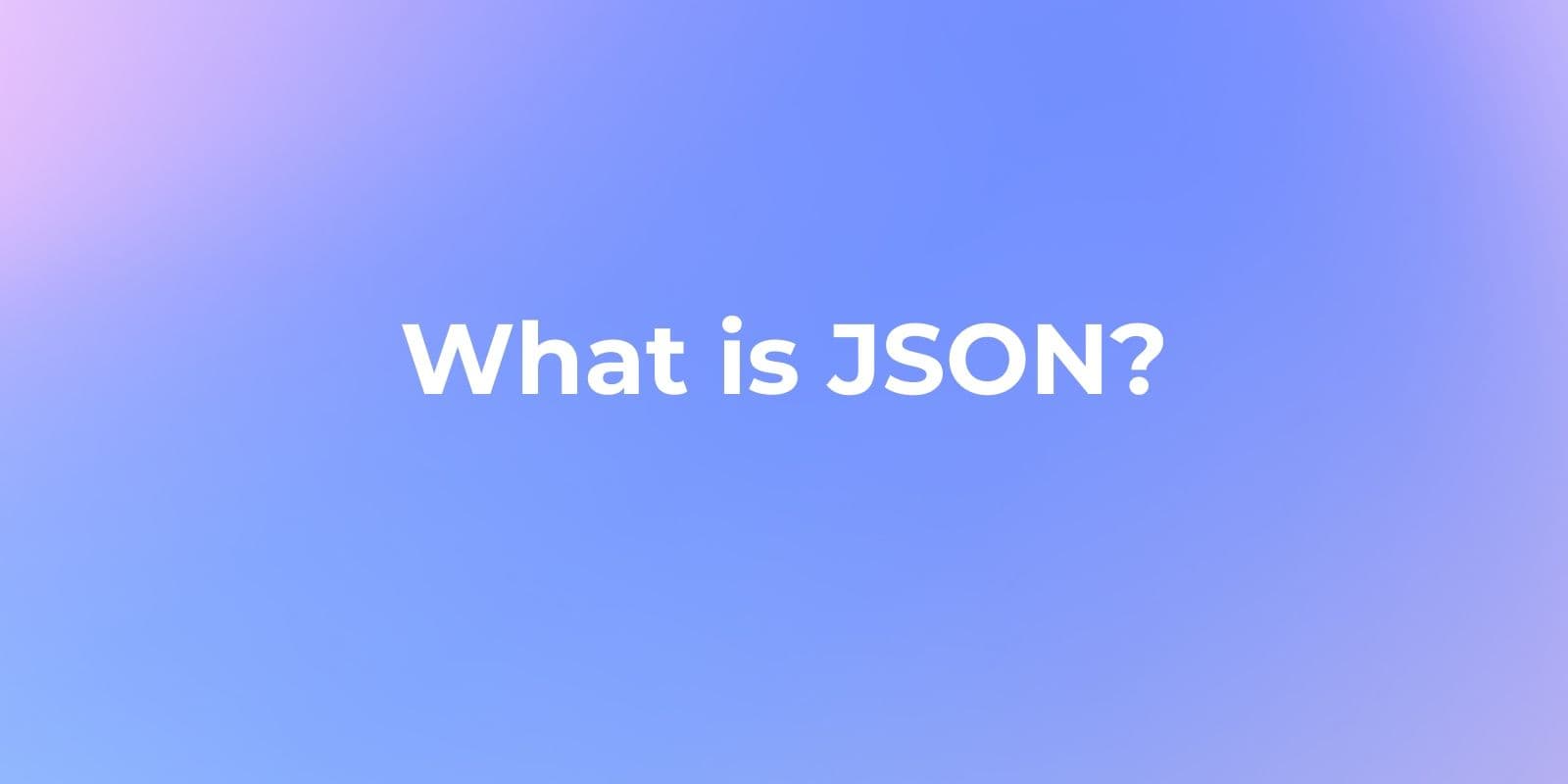
What is JSON? A Beginner's Guide to Understanding JSON
Many people mistakenly think of JSON (JavaScript Object Notation) as a programming language. In reality, JSON is a lightweight data interchange format that works seamlessly with most modern programming languages, such as Python, Java, PHP, and JavaScript. It is widely used for transmitting data in web applications due to its simplicity and efficiency.
What is JSON?
JSON is a text-based data format designed for exchanging information between systems. Its syntax closely resembles JavaScript objects, but JSON strictly consists of properties (key-value pairs) and does not support methods or functions.
Here’s what makes JSON stand out:
- Lightweight: JSON is smaller in size compared to formats like XML.
- Fast Parsing: Modern languages support easy parsing of JSON data, ensuring quicker access.
- Universally Compatible: JSON works natively with most programming languages, including JavaScript, Python, and PHP.
Why Do We Need JSON?
JSON is commonly used in web applications to facilitate fast and efficient communication between the server
and the client
. For example:
- Dynamic content updates based on user actions.
- Real-time suggestions, like personalized recommendations.
JSON Syntax and Structure
Understanding the basics of JSON syntax is essential for working with it effectively. Below is an example of data stored in JSON format:
{
"studentDetails": {
"name": "Joe",
"age": 16,
"dept": "computers",
"hobbies": ["dance", "books", "public speaking", "golf"],
"isClassLeader": false
}
}
Key Points of JSON Syntax:
- Curly Braces: JSON objects are enclosed within .
- Key-Value Pairs: Each item is written as "key": value.
- Quotes: Keys and string values are enclosed in double quotes ("). Numbers and booleans do not require quotes.
- Commas: Items are separated by commas, except after the last item.
- Arrays: Arrays are enclosed within [] and can contain objects, strings, numbers, or other arrays.
Features of JSON
- Nested Objects: JSON supports embedding objects and arrays within other objects.
- Flexible Schema: JSON data can be extended or modified easily without breaking the structure.
- No Functions: Unlike JavaScript objects, JSON does not accept functions or special types like
undefined
.
Example of a nested JSON object:
{
"continentName": "North America",
"area": 24.71,
"countries": [
{
"countryName": "Mexico",
"countryCode": "+52",
"location": "south of North America",
"languagesSpoken": ["Spanish", "Maya", "Nahuatl"]
}
]
}
Why Use JSON Over XML?
Before JSON, XML was the standard for transmitting data in web applications. However, JSON offers several advantages:
- Lightweight: JSON eliminates the extra tags used in XML.
- Faster Parsing: JSON is quicker to parse and process.
- Easier to Read: JSON’s name-value pair structure is more human-readable.
Real-World Applications of JSON
- Data Transfer in Web Applications: JSON is the primary format for client-server communication.
- Dynamic Web Content: JSON enables fast updates to web pages without requiring a full reload.
- MongoDB Integration: MongoDB stores data in BSON (Binary JSON), which is more efficient than JSON.
Working with JSON in JavaScript
JavaScript provides built-in methods to handle JSON data:
- Convert JS Objects to JSON: Use JSON.stringify().
- Parse JSON to JS Objects: Use JSON.parse().
Example:
let jsonData = {
"continentName": "North America",
"area": 24.71
};
// Convert object to JSON string
let jsonString = JSON.stringify(jsonData);
console.log(jsonString);
// Parse JSON string back to object
let parsedData = JSON.parse(jsonString);
console.log(parsedData.continentName); // Output: North America
JSON vs BSON
BSON, or Binary JSON, is a binary-encoded serialization format used by MongoDB. While JSON is great for lightweight data interchange, BSON offers:
- Faster queries.
- Space-efficient storage.
- Support for additional data types like binary data.
Conclusion
JSON is a must-know technology for modern developers. Its lightweight nature, simplicity, and compatibility with popular programming languages make it the ideal choice for data exchange in web applications. From dynamic websites to MongoDB integration, JSON continues to power seamless communication between clients and servers.
So, whether you’re building your first web app or optimizing server-client interactions, understanding JSON will give you a significant advantage!
Engineer solutions, innovate fearlessly, and build a better tomorrow today.