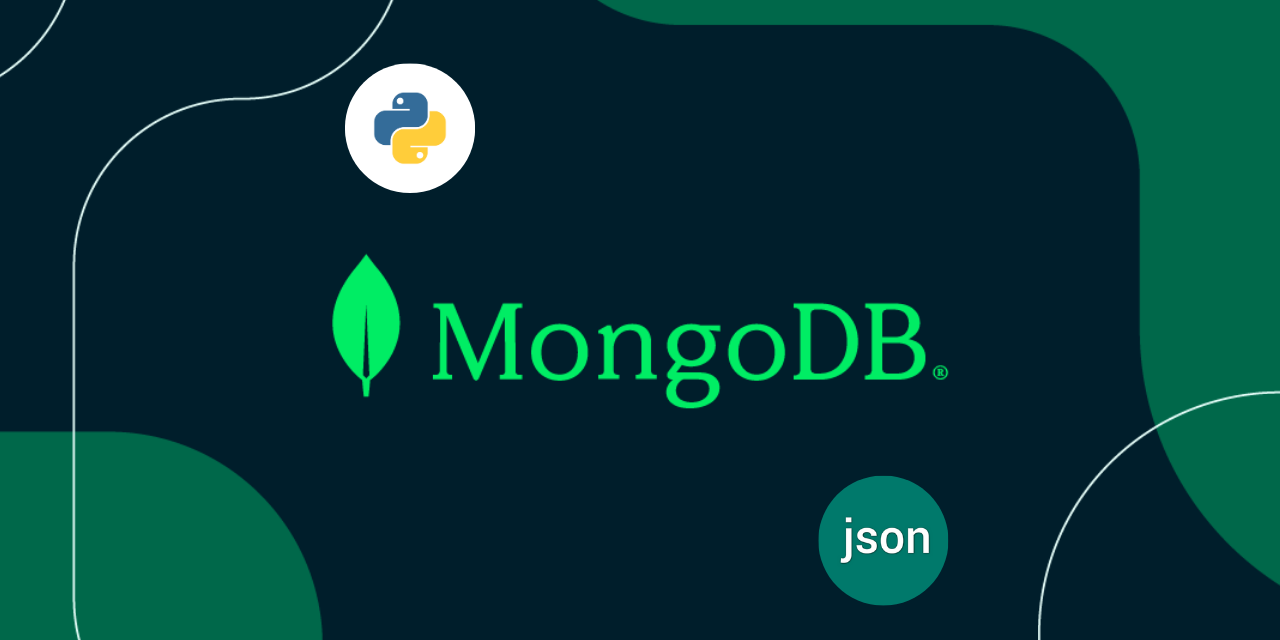
Backing Up JSON Files to MongoDB with Python
Efficient data management and storage are crucial for modern applications. Backing up JSON files to a database like MongoDB ensures scalability, reliability, and easy access to your data. In this guide, we’ll walk you through how to back up JSON files from a Python program to MongoDB.
Why Use MongoDB for JSON Backup?
MongoDB is a document-oriented NoSQL database designed to handle JSON-like documents. Here’s why it’s an excellent choice for backing up JSON files:
- Flexible Schema: MongoDB’s BSON format supports hierarchical and nested data.
- Scalable: Handles large datasets with ease.
- Integration: Works seamlessly with Python and other programming languages.
- Efficient Querying: Offers powerful querying capabilities for JSON data.
Prerequisites
Before getting started, ensure you have the following:
-
Python Installed: Version 3.7 or higher is recommended.
-
MongoDB: Install MongoDB locally or use a cloud-based solution like MongoDB Atlas.
-
Required Libraries: Install
pymongo
for Python to interact with MongoDB.pip install pymongo
Setting Up Your MongoDB Database
Start by creating a database and collection in MongoDB to store your JSON data. Here’s an example:
use jsonBackupDB;
db.createCollection("jsonFiles");
This creates a database named jsonBackupDB
with a collection called jsonFiles
.
Backing Up JSON Files in Python
The following steps will guide you through reading a JSON file and inserting its content into MongoDB.
1. Import Required Libraries
Start by importing the necessary Python libraries:
import json
from pymongo import MongoClient
2. Connect to MongoDB
Establish a connection to your MongoDB instance:
client = MongoClient("mongodb://localhost:27017/")
db = client["jsonBackupDB"]
collection = db["jsonFiles"]
Replace localhost
and 27017
with your MongoDB server address and port if different.
3. Load and Insert JSON Data
Load a JSON file and insert it into the database:
# Specify the path to your JSON file
json_file_path = "data.json"
# Open and load the JSON file
with open(json_file_path, "r") as file:
json_data = json.load(file)
# Insert data into MongoDB
if isinstance(json_data, list):
collection.insert_many(json_data) # For JSON arrays
else:
collection.insert_one(json_data) # For single JSON objects
print("Backup successful!")
4. Verify the Backup
You can verify the data insertion by querying the collection:
for document in collection.find():
print(document)
Error Handling and Logging
To ensure robustness, add error handling:
try:
with open(json_file_path, "r") as file:
json_data = json.load(file)
if isinstance(json_data, list):
collection.insert_many(json_data)
else:
collection.insert_one(json_data)
print("Backup successful!")
except Exception as e:
print(f"An error occurred: {e}")
Automating Backups
You can automate the backup process using the schedule
Python library:
Install the Library
pip install schedule
Automate the Script
Here’s an example of scheduling the backup script to run every day:
import schedule
import time
def backup_json():
try:
with open(json_file_path, "r") as file:
json_data = json.load(file)
if isinstance(json_data, list):
collection.insert_many(json_data)
else:
collection.insert_one(json_data)
print("Backup successful!")
except Exception as e:
print(f"An error occurred: {e}")
# Schedule the backup daily
schedule.every().day.at("01:00").do(backup_json)
while True:
schedule.run_pending()
time.sleep(1)
Conclusion
Backing up JSON files to MongoDB with Python is a straightforward process that ensures your data is secure and accessible. With MongoDB’s robust features and Python’s flexibility, you can scale your backup system as needed. Start implementing this today to safeguard your data efficiently!
Simplify today, secure tomorrow, and let innovation lead the way.